Recently, I've been playing with the new typescript feature of the Wix's editor and I must say I love it. It really helps write faster and catch errors early on. A must learn if you don't know it yet! I also realized it is a great solution for a problem I keep having when working on Wix's project:
How to correctly spell the property's name for my collection items?
When dealing with wixData API, I used to go back and forth between the content manager and my code simply to know how to spell a property correctly. For instance, what's the correct property for the custom profile image?
profilePicture
userProfile
userPicture
🤷
It really takes a toll on my productivity and I had to find a way to deal with that problem
JsDoc to the rescue
By using JsDoc, we can use Wix autocomplete to suggest the right spelling and even detect typos.
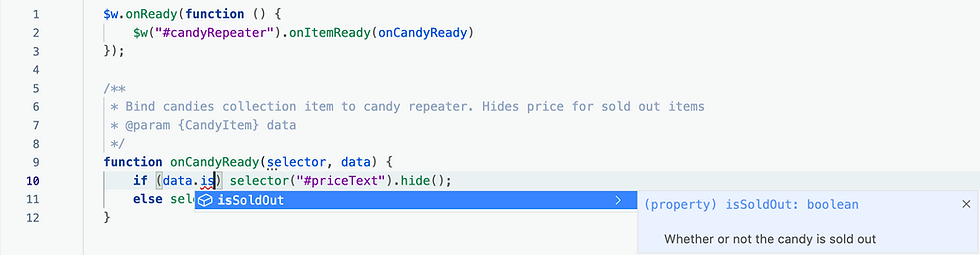
1. Defining your collections fields
The first step is to define the type of your collection
//public/collectionTypes.js
/**
* @typedef CollectionItem
* @property {string} _id - item unique identifier
* @property {Date} _createdDate - creation Date
* @property {Date} _updatedDate - last update Date
* @property {string} _owner - the item owner's _id
*/
/**
* @typedef Stores
* @property {string} managerEmail - manager email
* @property {string} propertieType - property type
* @property {string} image - store front photo
* @property {string} location - address(google format)
*/
/**
* @typedef Candies
* @property {string} brand - candy brand
* @property {number} sugar - number of gram of sugar per 100g
* @property {boolean} isSoldOut - Whether or not the candy is sold out
* @property {string} image - url of the candy's image
* @property {string} description - candy's description
* @property {string} priceFormatted - formatted price
* @property {number} price - price's value in USD
*/
/** @typedef {CollectionItem & Stores} StoreItem */
/** @typedef {CollectionItem & Candies} CandyItem */
2. Using your custom collection type
Simply define your variable's type and enjoy the greatness of autocomplete.
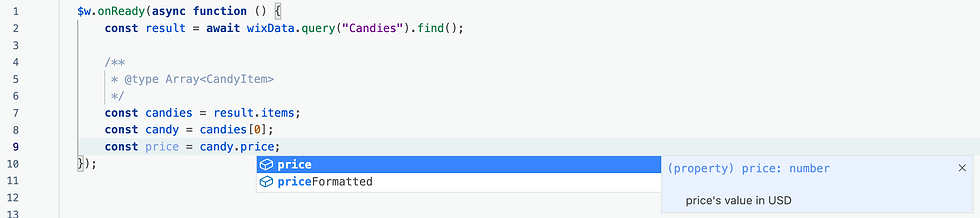
Benefits
On top of reducing the amount of time you spend in the content manager, it's a great monitoring tool that helps catch issues early.
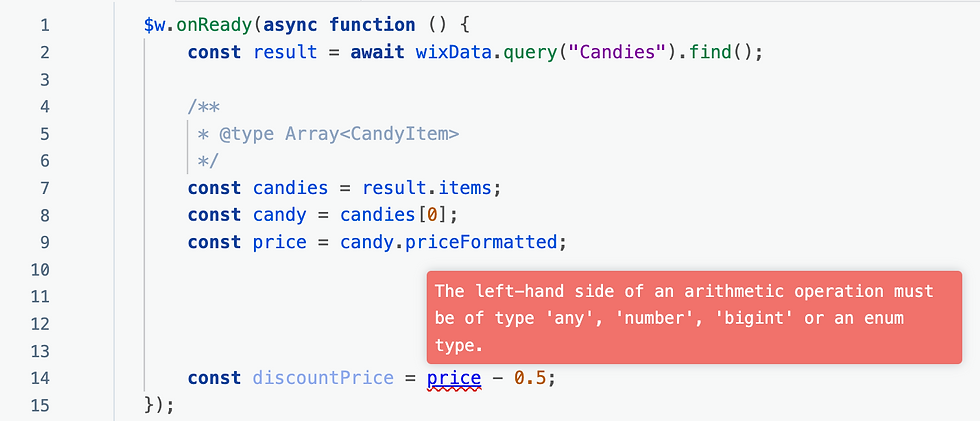
Velo detecting that we are trying to compute the discounted price on the formatted price instead of the price
It is also useful when you decide to change your collection structure; Once the changes to your collection are reflected in your JSDoc, all instances that use a deprecated property will be highlighted by the editor. This is handy to easily spot breaking changes in your code.
You can now lie back and enjoy your coding session knowing you won't have any more problems with your data field.
I hope this technique will be helpful and will improve the quality of your projects.
Let me know what you think of this technique and if you have any questions can contact me via the forum (@kentin)
I am using it now. I like the way you combined types! so much easier